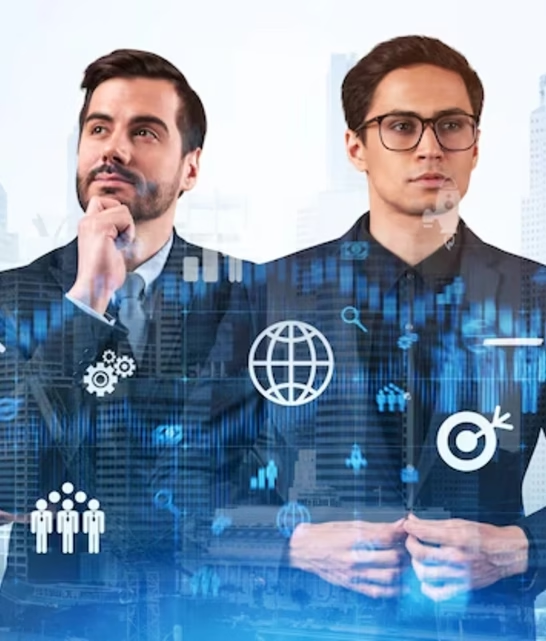
Building a web application requires various technology choices, and one of the most critical is selecting the right backend framework. Two of the most popular options for backend web development are Express and Django.
In this comprehensive guide, we’ll compare these two frameworks across 10 key factors to help you determine which is the better choice for your next web application project.
Let’s start with a quick introduction to both frameworks.
Express is a fast, minimalist web framework for Node.js. It provides a robust set of features for building single-page, multi-page, and hybrid web applications. Express is unopinionated, allowing developers to structure their projects however they want.
Some key features of Express include:
Express applications are written in JavaScript and can be used to build fullstack apps when paired with Node.js on the backend. Companies like Uber, IBM, PayPal, and LinkedIn use Express in production.
Django is a batteries-included, Python-based web framework that enables rapid development. It includes a host of out-of-the-box features like an ORM, admin interface, templating engine, etc.
Some key highlights of Django include:
Django follows the “Django way” which emphasizes explicitness over magic, dry principle, and overall simplicity. High-traffic sites like Instagram, Spotify, and Dropbox use Django successfully in production.
1. Performance
Performance is a critical factor when choosing a backend framework. Let’s see how Express and Django compare.
Express Performance
Express provides stellar performance thanks to its lightweight nature and being built on top of Node.js. Here are some key performance advantages of Express:
In benchmarks, an Express server can handle over 11k requests/sec which is significantly higher than other popular frameworks like Ruby on Rails. Overall, Express gives near real-time performance suitable for today’s demanding web and mobile apps.
Django Performance
Django uses a synchronous programming model which can impact its ability to handle very high concurrency. However, with the right optimizations, Django can deliver excellent performance:
While Django may not match Express in raw performance, it remains significantly faster than frameworks like Ruby on Rails. Optimizations can help it easily serve high-traffic websites. Overall, Django’s performance is more than adequate for most applications.
Verdict
For sheer performance with high concurrency loads, Express is the clear winner.
2. Ease of Use
Both Express and Django are designed to improve developer productivity. Let’s compare them on simplicity and ease of use.
Express Ease of Use
Express provides a minimalist web framework on top of Node.js. Here are some of its characteristics that impact ease of use:
The simplicity of Express makes it easy for beginners to pick up. The lack of strict rules also gives experienced developers a lot of flexibility.
Django Ease of Use
Django focuses heavily on rapid development and creator productivity:
The batteries-included nature of Django lets beginners be productive quickly. Django’s consistency also makes it easy for teams to collaborate efficiently.
Verdict
For beginners, Django’s higher level abstractions provide a slight edge. But Express offers more flexibility for advanced developers.
3. Scalability
The backend framework must provide good support for scaling an application to handle increasing traffic and data volumes.
Express Scalability
Express benefits from Node’s asynchronous, event-driven architecture to provide great scalability:
Its lightweight nature makes Express very scalable out of the box. Also, it imposes very few restrictions on application design, making it flexible.
Django Scalability
Django provides many features to allow applications to scale up smoothly:
Django powers very large sites dealing with millions of users and requests. With the right architecture, scaling limitations can be overcome.
Verdict
Express provides more innate scalability. But Django has the capability to scale large as well through deliberate design choices.
4. Reliability
Mission-critical web applications must have high reliability and uptime. Let’s see how Express and Django compare.
Express Reliability
Express promotes coding practices that improve reliability:
Overall, Express enables building systems to be resilient to failures through intentional design choices by developers.
Django Reliability
Django provides many features that directly improve application reliability:
The default architectural patterns promoted by Django directly boost reliability for the majority of applications.
Verdict
Django wins when it comes to reliability right out of the box. But Express offers building resilient systems through code.
5. Feature Set
The richness of features provided natively by the framework influences the code you have to write.
Express Features
As a minimalist framework, Express only provides essential web app building blocks:
All other functionality must be coded manually or installed via third-party modules. This allows keeping apps lightweight.
Django Features
In contrast, Django provides a very rich set of higher level features:
This significantly reduces repetitive code that developers need to build the basics of a web application.
Verdict
Django easily wins due to its batteries-included philosophy and extensive feature set.
6. Learning Curve
For developers new to the framework, the learning curve determines how fast they can become productive.
Express Learning Curve
For JavaScript developers, Express has a very gentle learning curve:
The only challenge may be getting comfortable with asynchronous JavaScript if coming from a synchronous language.
Django Learning Curve
For Python developers, Django has a medium learning curve:
However, Django’s consistency, documentation and structured approach helps overcome the initial learning curve.
Verdict
Express has a markedly shallower learning curve over Django.
7. Support & Community
An active community and availability of support resources drive framework adoption.
Express Community Support
As one of the most popular web frameworks, Express has exceptional community support:
Resources for guides, questions, training, etc. are freely available showing Express is here to stay.
Django Community Support
As one of the most popular Python frameworks, Django has stellar community support:
Django also enjoys strong community support rivaling that of Express and other leading frameworks.
Verdict
Both Express and Django have excellent community support so this factor is a tie.
8. Security
Backend frameworks provide protections against common web application security risks. Let’s compare how Express and Django handle security.
Express Security
Express provides minimal but extensible security:
The modular approach allows applying security judiciously based on specific risks rather than being restrictive.
Django Security
Django provides robust security features built-in:
Django has a strong focus on “secure by default” to prevent common flaws upfront.
Verdict
For security out of the box, Django easily wins over Express.
9. Database Support
The data persistence options supported by a framework are important for web applications. Let’s see how Express and Django compare.
Express Database Support
Being non-opinionated, Express supports integrating any database:
Flexibility to bring your own data persistence engine is a significant advantage of Express.
Django Database Support
Django provides built-in ORM with extensive database support:
The ORM provides convenience while handling much of the complexity transparently.
Verdict
Django wins for built-in ORM providing superb convenience and database support.
10. Testability
The ease of testing an application determines how quickly bugs can be caught. Let’s explore testing support in Express and Django.
Express Testability
Express promotes great testability through its design:
The minimalist structure of Express apps keeps test complexity in control.
Django Testability
Django considers testability a first class concern:
Django provides many utilities right out of the box to minimize the effort required to thoroughly test apps.
Verdict
Django once again wins out with bake-in features that enable writing comprehensive tests with minimal effort.
Let’s summarize how Express and Django compare across the different factors:
As you can see, both Express and Django have their relative strengths and weaknesses. Express shines when it comes to simplicity, scalability and blazing performance. Django excels at reliability, built-in features, database handling and testability.
The right choice depends ultimately on your specific needs and use case.
For smaller applications where simplicity and scalability are key priorities, Express may be the best fit. If you need a rich out-of-box feature set and don’t want to reinvent the wheel, Django can help accelerate development.
Both frameworks have strong corporate backing, community support and availability of complementary tools. So you can’t go wrong picking either Express or Django for your next web application!
TAV Tech Solutions is a leading software development company specializing in offering a complete range of software service and technology solutions across industry verticals. The company is known for its team of some of the best designers and developers in the business. Together they create highly intuitive and efficient apps that drive businesses in this digital world. The company is dedicated to creating digital solutions that align perfectly with the requirements of the end user. With several years of industry experience they have emerged as a trusted provider of top-notch software solutions at pocket-friendly prices. Their range of offerings not only empowers businesses but also delights users with an interactive experience.
Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur
Admin | Content Manager
Let’s connect and build innovative software solutions to unlock new revenue-earning opportunities for your venture